SPRING_DI
๐ SPRING
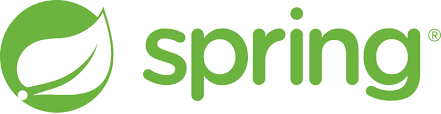
๐ Dependency Injection ( ์์กด์ฑ ์ฃผ์ )
Bean
Spring Bean์ ๊ธฐ๋ณธ์ ์ผ๋ก Singleton ์ผ๋ก ๋ง๋ค์ด์ง๋ค. ๊ทธ๋ฌ๋ฏ๋ก ์ปจํ
์ด๋๊ฐ ์ ๊ณตํ๋ ๋ชจ๋ ๋น์ instance๋ ๋์ผํ๋ค.
์ปจํ
์ด๋๊ฐ ํญ์ ์๋ก์ด instance๋ฅผ ๋ฐํํ๊ฒ ๋ง๋ค๊ณ ์ถ์ ๊ฒฝ์ฐ scope๋ฅผ prototype ์ผ๋ก ์ค์ ํด์ผํ๋ค.
@Service("memberService")
@Scope("singleton")
public class MemberServiceImpl implements MemberService{
@Override
public int registerMember(MemberDto memberDto){
return 0;
}
}
xml bean ์ค์ ( prototype )
<bean id="memberService" class="com.test.hello.service2.MemberServiceImpl" scope="prototype"/>
Bean์ ์์ฑ ๋ฒ์ ์ง์
- singleton
- spring container ๋น ํ๋์ instance bean๋ง ์์ฑ (default)
- prototype
- container์ bean์ ์์ฒญํ ๋๋ง๋ค ์๋ก์ด instance ์์ฑ
- request
- HTTP Request๋ณ๋ก ์๋ก์ด instance ์์ฑ
- session
- HTTP Session๋ณ๋ก ์๋ก์ด isntance ์์ฑ
bean ๋ฉํ ์ ๋ณด์ ํํ ๋ฐฉ์์๋ XML Document, Annotation, Java Code ๊ฐ ์๋๋ฐ ์ด๊ฒ๋ค์ ํตํด BeanDefinition meta data๊ฐ ๋์ด IoC Container์ ์ ์ก๋๋ค.
Spring Bean ์ค์
XML
- XML๋ฌธ์ ํํ๋ก ๋น์ ์ค์ ๋ฉํ ์ ๋ณด๋ฅผ ๊ธฐ์
-
ํ๊ทธ๋ฅผ ํตํด์ ์ธ๋ฐํ ์ ์ด ๊ฐ๋ฅ
servlet-context.xml
<bean id="memberDao" class="com.test.hello.dao.MemberDaoImpl" />
<bean id="memberService" class="com.test.hello.service2.MemberServiceImpl" scope="prototype" >
<property name="memberDao" ref="memberDao" />
</bean>
Annotation
- ์ดํ๋ฆฌ์ผ์ด์ ๊ท๋ชจ๊ฐ ์ปค์ง๊ณ ๋น์ ๊ฐ์๊ฐ ๋ง์์ง ์๋ก XML ํ์ผ ๊ด๋ฆฌํ๋ ๊ฒ์ด ๋ฒ๊ฑฐ๋กญ๋ค.
- ํน๋ณํ annotation์ ๋ถ์ฌํด ์ฃผ๋ฉด ์๋์ผ๋ก ๋น ๋ฑ๋ก์ด ๊ฐ๋ฅํ๋ค.
- โ์ค๋ธ์ ํธ ๋น ์ค์บ๋โ ๋ก โ๋น ์ค์บ๋โ์ ํตํด ์๋ ๋ฑ๋กํ๋ค.
- ๋น ์ค์บ๋๋ ๊ธฐ๋ณธ์ ์ผ๋ก ํด๋์ค ์ด๋ฆ์ ๋น์ ์์ด๋๋ก ์ฌ์ฉ
- ์ ํํ๋ ํด๋์ค ์ด๋ฆ์ ์ฒซ ๊ธ์๋ง ์๋ฌธ์๋ก ๋ฐ๊พผ ๊ฒ์ ์ด์ฉ
@Service
public class MemberServiceImpl implements MemberService{
// annotation์ ์ฌ์ฉํ ๋น ๋ฑ๋ก ( Autowired )
@Autowired
private MemberDao memberDao;
@Override
public int registerMember(MemberDto memberDto){
return memberDao.registerMember(memberDto);
}
}
Annotation์ผ๋ก ๋น์ ์ค์ ํ ๊ฒฝ์ฐ ๋ฐ๋์ component-scan์ ์ค์ ํด์ผ ํ๋ค.
servlet-context.xml
<context:component-scan base-package="com.test.hello.*" />
- Stereotype annotation ์ข
๋ฅ
- ๋น ์๋๋ฑ๋ก์ ์ฌ์ฉํ ์ ์๋ annotation
- ๋น ์๋์ธ์์ ์ํ annotation์ด ์ฌ๋ฌ๊ฐ์ง์ธ ์ด์
- ๊ณ์ธต๋ณ๋ก ๋น์ ํน์ฑ์ด๋ ์ข ๋ฅ๋ฅผ ๊ตฌ๋ถ
- AOP Pointcut ํํ์์ ์ฌ์ฉํ๋ฉด ํน์ annotation์ด ๋ฌ๋ฆฐ ํด๋์ค๋ง ์ค์ ๊ฐ๋ฅ
- ํน์ ๊ณ์ธต์ ๋น์ ๋ถ๊ฐ ๊ธฐ๋ฅ ๋ถ์ฌ
Stereotype | ์ ์ฉ ๋์ |
@Repository | Data Access Layer์ DAO ๋๋ Repository ํด๋์ค์ ์ฌ์ฉ DataAccessException ์๋๋ณํ๊ณผ ๊ฐ์ AOP์ ์ ์ฉ ๋์์ ์ ์ ํ๊ธฐ ์ํด ์ฌ์ฉ |
@Service | Service Layer์ ํด๋์ค์ ์ฌ์ฉ |
@Controller | Presentation Layer์ MVC Controller์ ์ฌ์ฉ ์คํ๋ง ์น ์๋ธ๋ฆฟ์ ์ํด ์น ์์ฒญ์ ์ฒ๋ฆฌํ๋ ์ปจํธ๋กค๋ฌ ๋น์ผ๋ก ์ ์ |
@Component | ์์ Layer ๊ตฌ๋ถ์ ์ ์ฉํ๊ธฐ ์ด๋ ค์ด ์ผ๋ฐ์ ์ธ ๊ฒฝ์ฐ์ ์ค์ |
Spring Bean์ ์๋ช ์ฃผ๊ธฐ
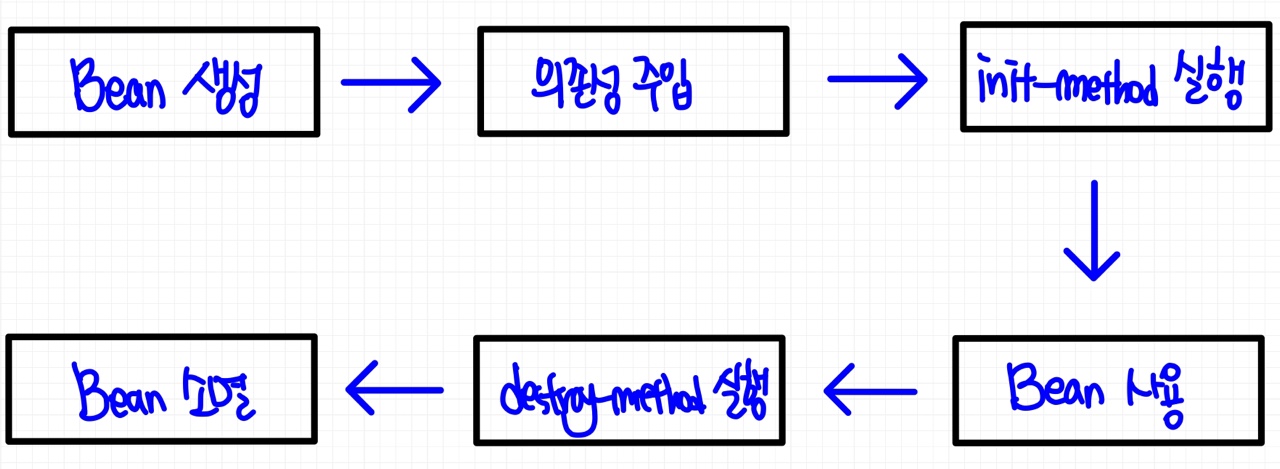
DI ( Dependency Injection )
- ์ธ๋ถ์์ ๋ ๊ฐ์ฒด ๊ฐ์ ๊ด๊ณ๋ฅผ ๊ฒฐ์ ํด์ฃผ๋ ๋์์ธ ํจํด
- ์ธํฐํ์ด์ค๋ฅผ ์ฌ์ด์ ๋ฌ์ ํด๋์ค ๋ ๋ฒจ์์๋ ์์กด๊ด๊ณ๊ฐ ๊ณ ์ ๋์ง ์๋๋ก ํ๊ณ ๋ฐํ์ ์์๋ ๊ด๊ณ๋ฅผ ๋ค์ด๋๋ฏนํ๊ฒ ์ฃผ์
- ์ ์ฐ์ฑ ํ๋ณด
-
๋์จํ ๊ฒฐํฉ ( loose coupling ) ์ ์ฃผ์ ๊ฐ์
- ๊ฐ์ฒด๋ ์ธํฐํ์ด์ค์ ์ํ ์์กด ๊ด๊ณ๋ง์ ์๊ณ ์์ผ๋ฉฐ, ์ด ์์กด ๊ด๊ณ๋ฅผ ๊ตฌํ ํด๋์ค์ ๋ํ ์ฐจ์ด๋ฅผ ๋ชจ๋ฅธ์ฑ ์๋ก ๋ค๋ฅธ ๊ตฌํ์ผ๋ก ๋์ฒด๊ฐ ๊ฐ๋ฅํ๋ค.
- ๊ฐ์ฒด ๊ฐ์ ์์กด๊ด๊ณ๋ฅผ ์์ ์ด ์๋ ์ธ๋ถ์ ์กฐ๋ฆฝ๊ธฐ๊ฐ ์ํ
-
Spring Container๊ฐ DI ์กฐ๋ฆฝ๊ธฐ๋ฅผ ์ ๊ณต
- Spring ์ค์ ํ์ผ์ ํตํ์ฌ ๊ฐ์ฒด ๊ฐ์ ์์กด๊ด๊ณ๋ฅผ ์ค์
- Spring Container๊ฐ ์ ๊ณตํ๋ API๋ฅผ ์ด์ฉํด ๊ฐ์ฒด๋ฅผ ์ฌ์ฉ
-
Spring Container๊ฐ DI ์กฐ๋ฆฝ๊ธฐ๋ฅผ ์ ๊ณต
DI ( Dependency Injection ) spring ์ค์
Spring ์ค์ - xml
xml ๋ฌธ์ ์ด์ฉ
- Application์์ ์ฌ์ฉํ Spring ์์๋ค์ ์ค์ ํ๋ ํ์ผ
- Spring Container๋ ์ค์ ํ์ผ์ ์ค์ ๋ ๋ด์ฉ์ ์ฝ์ด Application์์ ํ์ํ ๊ธฐ๋ฅ๋ค์ ์ ๊ณต
- Root tag๋
- ํ์ผ๋ช ์ ์๊ด ์๋ค.
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
๊ธฐ๋ณธ ์ค์ - bean ๊ฐ์ฒด ์์ฑ ๋ฐ ์ฃผ์
- ์ฃผ์
ํ ๊ฐ์ฒด๋ฅผ ์ค์ ํ์ผ์ ์ค์
-
: ์คํ๋ง ์ปจํ ์ด๋๊ฐ ๊ด๋ฆฌํ Bean๊ฐ์ฒด๋ฅผ ์ค์
-
- ๊ธฐ๋ณธ ์์ฑ
- name : ์ฃผ์ ๋ฐ์ ๊ณณ์์ ํธ์ถ ํ ์ด๋ฆ ์ค์
- id : ์ฃผ์ ๋ฐ์ ๊ณณ์์ ํธ์ถ ํ ์ด๋ฆ ์ค์ ( ์ ์ผ ๊ฐ )
- class : ์ฃผ์ ํ ๊ฐ์ฒด์ ํด๋์ค
- factory-method : Singleton ํจํด์ผ๋ก ์์ฑ๋ ๊ฐ์ฒด์ factory ๋ฉ์๋ ํธ์ถ
- ref : ์ฐธ์กฐ๋ฐ์ ํด๋์ค์ id๋ช
applicationContext.xml
<bean id="memberDao" class="com.test.hello.dao.MemberDaoImpl" />
<bean id="memberService" class="com.test.hello.service2.MemberServiceImpl" scope="prototype" >
<property name="memberDao" ref="memberDao" />
</bean>
๊ธฐ๋ณธ ์ค์ - bean ๊ฐ์ฒด ์ป๊ธฐ
- ์ค์ ํ์ผ์ ์ค์ ํ bean์ Container๊ฐ ์ ๊ณตํ๋ ์ฃผ์ ๊ธฐ ์ญํ ์ api๋ฅผ ํตํด ์ฃผ์ ๋ฐ๋๋ค.
java file
ApplicationContext context = new ClassPathXmlApplicationContext("com/test/hello/controller4/applicationContext.xml");
CommonService memberService = context.getBean("memberService", MemberService.class);
CommonService adminService = context.getBean("adminService", AdminService.class);
Spring ์ค์ - annotation
Annotation : ๋ฉค๋ฒ๋ณ์์ ์ง์ ์ ์ํ๋ ๊ฒฝ์ฐ setter method๋ฅผ ๋ง๋ค์ง ์์๋ ๋จ
@Resource, @Autowired, @Inject๊ฐ ์๋๋ฐ @Autowired๋ฅผ ์ฃผ๋ก ์ฌ์ฉํ๋ค.
@Autowired๋ Spring Framework์์ ์ง์ํ๋ Dependency ์ ์ ์ฉ๋์ Annotation์ผ๋ก, Spring Framework์ ์ข
์์ ์ด๊ธด ํ์ง๋ง ์ ๋ฐํ Dependency Injection์ด ํ์ํ ๊ฒฝ์ฐ์ ์ ์ฉํ๋ค.
์ฌ์ฉ๋ฒ์ ์์ฑ์์ ๋ฑ๋ก ํ๊ณ ๋์ผํ ํ์
์ bean์ด ์ฌ๋ฌ ๊ฐ์ผ ๊ฒฝ์ฐ์๋ @Qualifier(โnameโ) ์ผ๋ก ์๋ณํ๋ค.
public class MemberServiceImpl implements MemberService{
@Autowired
@Qualifier("mdao") // ๋์ผ ํ์
์ด ์ฌ๋ฌ๊ฐ ์ผ ๊ฒฝ์ฐ
MemberDao memberDao;
...
}