JAVASCRIPT_DOM
๐ JAVASCRIPT
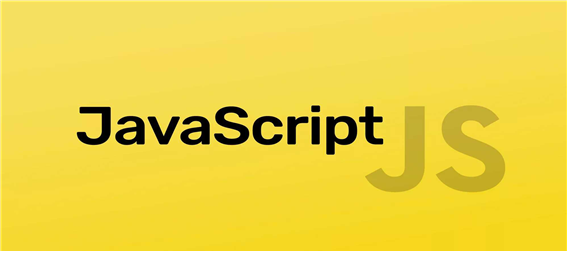
๐ DOM
DOM ์ด๋?
- DOM ( Document Object Model ) ์ HTML๊ณผ XML ๋ฌธ์์ ๊ตฌ์กฐ๋ฅผ ์ ์ํ๋ API ์ ๊ณตํ๋ค.
- DOM์ ๋ฌธ์ ์์ ์งํฉ์ ํธ๋ฆฌ ํํ์ ๊ณ์ธต ๊ตฌ์กฐ๋ก HTML์ ํํํ๋ค.
- HTML ๊ณ์ธต ๊ตฌ์กฐ์ ์ ์ผ ์์๋ document ๋ ธ๋๊ฐ ์๋ค.
- ๊ทธ ์๋๋ก HTML ํ๊ทธ๋ ์์๋ค์ ํฌํจํ๋ ๋ ธ๋์ ๋ฌธ์์ด์ ํฌํจํ๋ ๋ ธ๋๊ฐ ์๋ค.
<!DOCTYPE html>
<html>
<head>
<title>์ ๋ชฉ ์
๋๋ค.</title>
</head>
<body>
<h3>h3 ๋ฌธ์์ด์
๋๋ค.</h3>
<h2>h2 ๋ฌธ์์ด์
๋๋ค.</h2>
</body>
</html>
DOM ๋ฌธ์ ๊ณ์ธต ๊ตฌ์กฐ
- Document๋ HTML๋๋ XML ๋ฌธ์๋ฅผ ํํํ๋ค.
- HTMLDocument๋ HTML ๋ฌธ์์ ์์๋ง์ ํํ
- HTMLElement์ ํ์ ํ์ ์ HTML ๋จ์ผ ์์๋ ์์ ์งํฉ์ ์์ฑ์ ํด๋นํ๋ JavaScript ํ๋กํผํฐ๋ฅผ ์ ์ํ๋ค.
- Comment ๋ ธ๋๋ HTML์ด๋ XML์ ์ฃผ์์ ํํํ๋ค.
๋ฌธ์ ๊ฐ์ฒด ๋ง๋ค๊ธฐ
๋ฌธ์ ๊ฐ์ฒด
-
createElement(tagName)
element node๋ฅผ ์์ฑํ๋ค. -
createTagNode(text)
text node๋ฅผ ์์ฑํ๋ค. -
appendChild(node)
๊ฐ์ฒด์ node๋ฅผ child๋ก ์ถ๊ฐํ๋ค.
// ์๋์ฐ ํ์ด์ง๊ฐ ์ด๋ฆฌ๋ฉด ํจ์ ์คํ ํ๋ค๋ ์๋ฏธ์ด๋ค.
window.onload = function(){
var title = document.createElement('h2');
var msg = document.createTagNode('Hello !!');
// text node๋ฅผ element node์ ์ถ๊ฐํ๋ค.
title.appendChild(msg);
// title์ body์ ์ถ๊ฐํ๋ค.
document.body.appendChild(title);
}
-> ์ด ๊ฒฐ๊ณผ๋ body์ h2 ์์ฑ์ "Hello !!"๊ฐ ์ถ๋ ฅ ๋๋ค.
๊ฐ์ฒด์ ์์ฑ ์ค์
-
setAttribute(name, value)
๊ฐ์ฒด์ ์์ฑ์ ์ง์ ํ๋ค. -
getAttribute(name)
๊ฐ์ฒด์ ์์ฑ๊ฐ์ ๊ฐ์ ธ์จ๋ค.
// ์๋์ฐ ํ์ด์ง๊ฐ ์ด๋ฆฌ๋ฉด ํจ์ ์คํ ํ๋ค๋ ์๋ฏธ์ด๋ค.
// ์ด ๋ฐฉ๋ฒ์ด ๊ฐ์ฅ ๊ฐ๋จํ ๋ฐฉ๋ฒ์ด์ง๋ง ์น ํ์ค์ด๋ ์น ๋ธ๋ผ์ฐ์ ๊ฐ ์ง์ํ๋ ํ๊ทธ์ ์์ฑ๋ง ๊ฐ๋ฅํ๋ค !!
window.onload = function () {
var profile = document.createElement("img");
profile.src = "profile.png";
profile.width = 50;
profile.height = 100;
document.body.appendChild(profile);
};
// ์ง์ํ์ง ์๋ ํ๊ทธ์ ์์ฑ๋ ๊ฐ๋ฅํ ๋ฐฉ๋ฒ !!
window.onload = function () {
var profile = document.createElement("img");
profile.setAttribute("src", "profile.png");
profile.setAttribute("width", 50);
profile.setAttribute("height", 100);
profile.setAttribute("data-content", "๋ด์ฌ์ง");
document.body.appendChild(profile);
};
innerHTML & innerText
-
innerHTML
๋ฌธ์์ด์ HTML ํ๊ทธ๋ก ์ฝ์ ํ๋ค. -
innerText
๋ฌธ์์ด์ text node๋ก ์ฝ์ ํ๋ค.
window.onload = function(){
var html = document.getElementById('divHtml');
var text = document.getElementById('divText');
html.innerHTML = "<h2> Hello !! </h2>"
text.innerText = "<h2> Hello !! </h2>"
-> html์ html ์์๋๋ก ์ถ๋ ฅ๋์ง๋ง ( Hello !! )
-> text๋ ๊ทธ๋๋ก ์ถ๋ ฅ ๋๋ค ( <h2> Hello !! </h2>)
}
๋ฌธ์ ๊ฐ์ฒด ๊ฐ์ ธ์ค๊ธฐ
๊ฐ์ฒด ๊ฐ์ ธ์ค๊ธฐ
- getElementById(id)
ํ๊ทธ์ id ์์ฑ์ด id ์ ์ผ์นํ๋ element ๊ฐ์ฒด ์ป๊ธฐ
<script type="text/javascript">
window.onload = function(){
var msg = document.getElementById('head');
msg.innerHTML = 'hi !!!';
};
</script>
</head>
<body>
<h2 id='head'>hello!!</h2>
</body>
-> hi !!! ๊ฐ ์ถ๋ ฅ๋๋ค.
- getElementsByClassName(classname)
ํ๊ทธ์ classname ์์ฑ์ด classname ๊ณผ ์ผ์นํ๋ element ๋ฐฐ์ด ์ป๊ธฐ
<script type="text/javascript">
window.onload = function(){
var a = document.getElementsByClassName('class');
a.style.backcolor = 'red';
};
</script>
</head>
<body>
<h2 class='class'>hello!!</h2>
</body>
-> hello!! ๊ฐ ๋ฐฐ๊ฒฝ์ด ๋นจ๊ฐ์์ผ๋ก ์ถ๋ ฅ๋๋ค.
- getElementsByTagName(tagname)
ํ๊ทธ์ด๋ฆ์ด tagname ๊ณผ ์ผ์นํ๋ element ๋ฐฐ์ด ์ป๊ธฐ
<script type="text/javascript">
window.onload = function(){
var a = document.getElementsByTagName('h2');
a.style.backcolor = 'red';
};
</script>
</head>
<body>
<h2>hello!!</h2>
</body>
-> hello!! ๊ฐ ๋ฐฐ๊ฒฝ์ด ๋นจ๊ฐ์์ผ๋ก ์ถ๋ ฅ๋๋ค.
-
getElementsByName(name)
ํ๊ทธ์ name ์์ฑ์ด name ๊ณผ ์ผ์นํ๋ element ๋ฐฐ์ด ์ป๊ธฐ -
querySelector(selector)
selector์ ์ผ์นํ๋ ์ฒซ๋ฒ์งธ element ๊ฐ์ฒด ์ป๊ธฐ
class ๋ querySelector(โ.classNameโ);
id ๋ querySelector(โ#IDNameโ);
๋๋ฌธ์๋ก ๋ฐ๋ ๋ฐฉ๋ฒ์ querySelector(โh2[id=head]โ); ์ด ์๋ค. ์ด ๊ฒฝ์ฐ๋ ์ด๋ฆ์ ์ธ๋๋ฐ๊ฐ ์๋ ๊ฒฝ์ฐ์ ์ฌ์ฉํ๋ค.
<script type="text/javascript">
window.onload = function(){
var msg = document.querySelector('#head');
msg.innerHTML = 'hi !!!';
};
</script>
</head>
<body>
<h2 id="head">hello!!</h2>
</body>
-> hi !!! ๊ฐ ์ถ๋ ฅ๋๋ค.
- querySelectorAll(selector)
selector์ ์ผ์นํ๋ ๋ชจ๋ element ๋ฐฐ์ด ์ป๊ธฐ
<script type="text/javascript">
window.onload = function(){
var a = document.querySelectorAll('.class');
a.style.backcolor = 'red';
};
</script>
</head>
<body>
<h2 class="class">hello 1</h2>
<h2 class="class">hello 2</h2>
<h2 class="class">hello 3</h2>
</body>
-> hello 1, hello 2, hello 3 ์ด ๋ฐฐ๊ฒฝ์ด ๋นจ๊ฐ์์ผ๋ก ์ถ๋ ฅ๋๋ค.
๋ฌธ์ ๊ฐ์ฒด ์ ๊ฑฐํ๊ธฐ
๊ฐ์ฒด ์ ๊ฑฐ
-
removeChild(childnode)
๊ฐ์ฒด์ ์์ ๋ ธ๋๋ฅผ ์ ๊ฑฐํ๋ค.
<head>
<script type="text/javascript">
window.onload = function () {
var input4 = document.querySelector("#input4");
document.body.removeChild(input4);
};
</script>
</head>
<body>
<h2 id="input1">input1</h2>
<h2 id="input2">input2</h2>
<h2 id="input3">input3</h2>
<h2 id="input4">input4</h2>
</body>
// input4 ๋ ์ถ๋ ฅ๋์ง ์๋๋ค
DOM ๋ง๋ฌด๋ฆฌ
JavaScript์์ ์ค์ํ ๋ถ๋ถ์ธ DOM ์ ๋ํด์ ๊ณต๋ถ๋ฅผ ํ์๋ค.
HTML์์ ์์ฑํ ์ฝ๋๋ฅผ ๋์ ์ผ๋ก ์คํํ๋ ค๋ฉด id๋ class๋ฅผ ๊ฐ์ ธ์์ DOM์ API๋ฅผ ์ด์ฉํด ์คํํด์ค๋ค.
๊ทธ๋ฆฌ๊ณ ๊ฐ์ฒด๋ฅผ ๊ฐ์ ธ์ฌ ๋ getElement ์ querySelect ์ค ํ๋๋ฅผ ์ ํํ๋ ๊ฒ์ด ๋์๋ฐ ๊ต์๋์ querySelect๋ฅผ ์ถ์ฒํด ์ฃผ์
จ๋ค ใ
ใ
๊ฐ์ฒด๋ฅผ ๊ฐ์ ธ์์ ๋์์ ์ํค๊ฑฐ๋ ๊ฐ์ฒด์ ์์ฑ์ ์์ฑ, ๋ณ๊ฒฝํ๊ฑฐ๋ ์ฌ๋ฌ๊ฐ์ง๋ฅผ DOM์ ํตํด ํ๋๊น DOM API์ ๋ํด ์ ์์ ๋์ด์ผ ํ ๊ฒ ๊ฐ๋ค !!