BOJ_G4_1197
๐ [G4_1197] ์ต์ ์คํจ๋ ํธ๋ฆฌ
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.StringTokenizer;
public class Main {
static class Edge implements Comparable<Edge>{
int from;
int to;
int weight;
public Edge(int from, int to, int weight) {
super();
this.from = from;
this.to = to;
this.weight = weight;
}
// ์ค๋ฆ์ฐจ์ ์ ๋ ฌ
@Override
public int compareTo(Edge o) {
return o.weight >= this.weight ? -1:1;
}
}
static int N;
static int[] parents;
static Edge[] edgeList;
// ๋จ์ ์งํฉ ์์ฑ
public static void makeSet(){
parents = new int[N];
// ์์ ์ ๋ถ๋ชจ๋
ธ๋๋ฅผ ์์ ์ ๊ฐ์ผ๋ก ์ธํ
for(int i=0; i<N; i++){
parents[i]= i;
}
}
// a์ ์งํฉ ์ฐพ๊ธฐ : a์ ๋ํ์ ์ฐพ๊ธฐ
public static int findSet(int a){
if(a == parents[a]) return a;
// ๋์ ๋ถ๋ชจ์ ๋ถ๋ชจ์ ๋ถ๋ชจ์ .. ๋ฅผ ์ฐพ์ผ๋ฌ ๊ฐ๋ค
// path compression
return parents[a] = findSet(parents[a]);
}
// a,b ๋ ์งํฉ ํฉ์น๊ธฐ
public static boolean union(int a, int b){
int aRoot = findSet(a);
int bRoot = findSet(b);
// ๋ง์ฝ ๊ฐ์ผ๋ฉด ํฉ์น ํ์๊ฐ ์๋ค
if(aRoot == bRoot) return false;
// ์งฑ๋ผ๋ฆฌ ํฉ์ณ์ผ ํ๋ค
parents[bRoot] = aRoot;
return true;
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st= new StringTokenizer(br.readLine()," ");
N = Integer.parseInt(st.nextToken());
int E = Integer.parseInt(st.nextToken());
edgeList = new Edge[E];
for(int i=0; i<E; i++){
st = new StringTokenizer(br.readLine()," ");
int from = Integer.parseInt(st.nextToken());
int to = Integer.parseInt(st.nextToken());
int weight = Integer.parseInt(st.nextToken());
edgeList[i] = new Edge(from-1, to-1, weight);
}
// ๊ฐ์ ๋น์ฉ์ ์ค๋ฆ์ฐจ์ ์ ๋ ฌ
Arrays.sort(edgeList);
makeSet();
// ๊ฒฐ๊ณผ ๋น์ฉ
int result = 0;
// ์นด์ดํ
int cnt = 0;
for(Edge edge : edgeList){
if(union(edge.from, edge.to)){
result += edge.weight;
if(++cnt == N-1) break;
}
}
System.out.println(result);
}
}
๐ค ๋์ ์๊ฐ
MST์ ๊ธฐ๋ณธ ๋ฌธ์ ์ด๋ค.
๋๋ MST์ ํฌ๋ฃจ์ค์นผ ์๊ณ ๋ฆฌ์ฆ์ ์ฌ์ฉํด์ ํด๊ฒฐํ๋ค.
์ฌ๊ธฐ์ ์์ ๊ฐ์ค์น๊น์ง ์๊ธฐ ๋๋ฌธ์ comparable ๋ถ๋ถ์ ์์๊น์ง ๊ณ ๋ คํด์ ์ฒ๋ฆฌํด์ฃผ์๋ค.
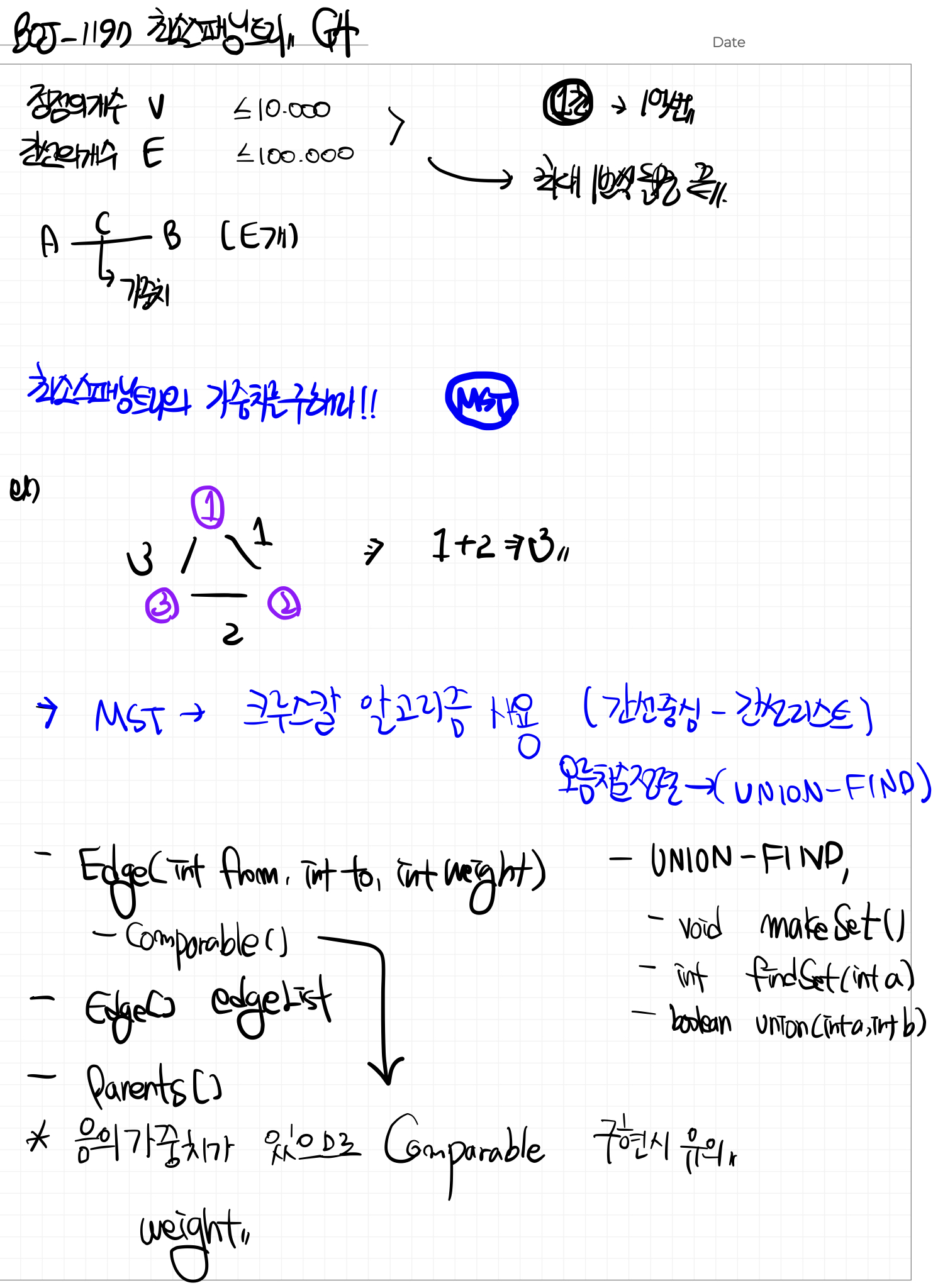